
May 31, 2023 10:10 by
Peter
Action filter in MVC allows us to manage situations in which we wish to perform an operation prior to and following the execution of a controller action. We create a custom class that inherits the FilterAttribute class and implements the IActionFilter interface for this purpose. After creating the filter, we merely assign the class name to the controller as an attribute.
In this case, the FilterAttribute class allows the class to be used as an attribute, and the IActionFilter interface contains two methods titled OnActionExecuting and OnActionExecuted. OnActionExecuting is executed before the controller method, while OnActionExecuted is summoned after the controller method has been executed. This technique is extremely useful for archiving purposes. So let's examine how we can utilize this filter.
Let's begin by adding the MyActionFilter.cs class. Derive this class now from the FilterAttribute and the IActionFilter interfaces. Incorporate your custom logic within the OnActionExecuting and OnActionExecuted methods.Consequently, the code will appear as shown below.
public class MyActionFilter : FilterAttribute, IActionFilter
{
public void OnActionExecuted(ActionExecutedContext filterContext)
{
//Fires after the method is executed
}
public void OnActionExecuting(ActionExecutingContext filterContext)
{
//Fires before the action is executed
}
}
Simply, apply the class as an attribute on the controller. Add debuggers on both the methods as well as the controller method.
public class HomeController : Controller
{
[MyActionFilter]
public ActionResult Index()
{
return View();
}
public ActionResult About()
{
ViewBag.Message = "Your application description page.";
return View();
}
public ActionResult Contact()
{
ViewBag.Message = "Your contact page.";
return View();
}
}
Run the Application and debug step by step to see the order of execution of the methods. First, the OnActionExecuting will be executed, then the controller method and finally the OnActionExecuted method.
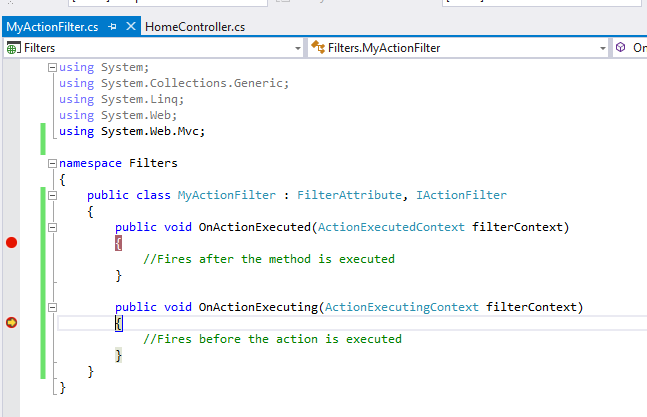
I hope you enjoyed reading it. Happy coding.