In this tutorial, we will discuss about how you can increase the performance of website using ASP.NET MVC.
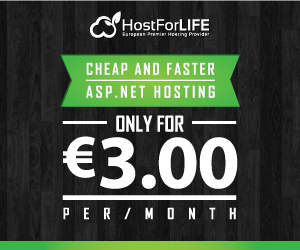
1. Remove Unused view engines
protected void Application_Start()
{
ViewEngines.Engines.Clear();
ViewEngines.Engines.Add(new RazorViewEngine());
}
2. Deploying Production Code in Release Mode
Make sure your production application always runs in release mode in the web.config
<compilation debug=”false”></compilation>
<configuration> <system.web> <deployment retail=”true”></deployment> </system.web> </configuration>
3. Use OutputCacheAttribute When Appropriate
MVC will not do any View Look-up Caching if you are running your application in Debug Mode
[OutputCache(VaryByParam = "none", Duration = 3600)]
public ActionResult Categories()
{
return View(new Categories());
}
4. Use HTTP Compression
Add gzip (HTTP compression) and static cache (images, css, …) in your web.config:
<system.webserver><urlcompression dodynamiccompression=”true” dostaticcompression=”true” dynamiccompressionbeforecache=”true”></urlcompression>
</system.webserver>
5. Add an Expires or a Cache-Control Header
<configuration><system.webServer>
<staticContent>
<clientCache cacheControlMode=”UseExpires”
httpExpires=”Mon, 06 May 2013 00:00:00 GMT” />
</staticContent>
</system.webServer>
</configuration>
6. Uncontrolled Actions
protected override void HandleUnknownAction(string actionName)
{
RedirectToAction("Index").ExecuteResult(this.ControllerContext);
}
7. Other Ways
- Avoid passing null models to views
- Remove unused HTTP Modules
- Put repetitive code inside your PartialViews
- Put Stylesheets at the Top
- Put Scripts at the Bottom
- Make JavaScript and CSS External
- Minify JavaScript and CSS
- Remove Duplicate Scripts
- No 404s
- Avoid Empty Image src
- Use a Content Delivery Network
- Use either Microsoft, Google CDN for referencing the Javascript or Css libraries
- Use GET for AJAX Requests
- Optimize Images
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
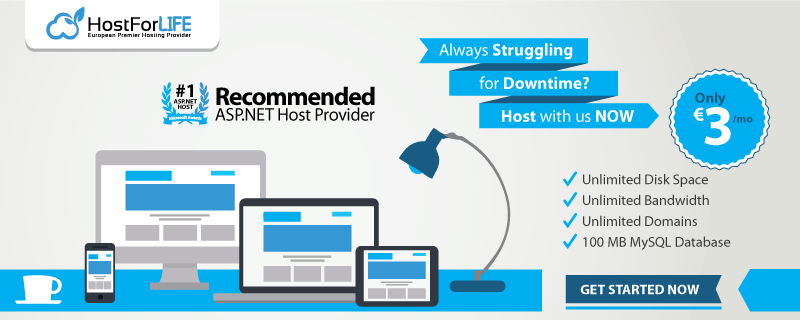