In this post, I will create simple mail helper class for sending emails in ASP.NET MVC using C#.
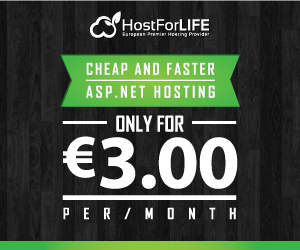
IMPLEMENTATION
Step 1
Create a class name MailHelper and the add the following code:
public class MailHelper
{
private const int Timeout = 180000;
private readonly string _host;
private readonly int _port;
private readonly string _user;
private readonly string _pass;
private readonly bool _ssl;
public string Sender { get; set; }
public string Recipient { get; set; }
public string RecipientCC { get; set; }
public string Subject { get; set; }
public string Body { get; set; }
public string AttachmentFile { get; set; }
public MailHelper()
{
//MailServer - Represents the SMTP Server
_host = ConfigurationManager.AppSettings["MailServer"];
//Port- Represents the port number
_port = int.Parse(ConfigurationManager.AppSettings["Port"]);
//MailAuthUser and MailAuthPass - Used for Authentication for sending email
_user = ConfigurationManager.AppSettings["MailAuthUser"];
_pass = ConfigurationManager.AppSettings["MailAuthPass"];
_ssl = Convert.ToBoolean(ConfigurationManager.AppSettings["EnableSSL"]);
}
public void Send()
{
try
{
// We do not catch the error here... let it pass direct to the caller
Attachment att = null;
var message = new MailMessage(Sender, Recipient, Subject, Body) { IsBodyHtml = true };
if (RecipientCC != null)
{
message.Bcc.Add(RecipientCC);
}
var smtp = new SmtpClient(_host, _port);
if (!String.IsNullOrEmpty(AttachmentFile))
{
if (File.Exists(AttachmentFile))
{
att = new Attachment(AttachmentFile);
message.Attachments.Add(att);
}
}
if (_user.Length > 0 && _pass.Length > 0)
{
smtp.UseDefaultCredentials = false;
smtp.Credentials = new NetworkCredential(_user, _pass);
smtp.EnableSsl = _ssl;
}
smtp.Send(message);
if (att != null)
att.Dispose();
message.Dispose();
smtp.Dispose();
}
catch (Exception ex)
{
}
}
}
Step 2
Place the following code in the app settings of your application:
appSettings>
<add key=”MailServer” value=”smtp.gmail.com”/>
<add key=”Port” value=”587″/>
<add key=”EnableSSL” value=”true”/>
<add key=”EmailFromAddress” value=”[email protected]”/>
<add key=”MailAuthUser” value=”[email protected]”/>
<add key=”MailAuthPass” value=”xxxxxxxx”/>
</appSettings>
Step 3
If you don’t have authentication for sending emails you can pass the emtpy string in MailAuthUser and MailAuthPass.
<appSettings>
<add key=”MailServer” value=”smtp.gmail.com”/>
<add key=”Port” value=”587″/>
<add key=”EnableSSL” value=”true”/>
<add key=”EmailFromAddress” value=”[email protected]”/>
<add key=”MailAuthUser” value=””/>
<add key=”MailAuthPass” value=””/>
</appSettings>
USAGE
Add the following code snippet in your controller to call Mail Helper class for sending emails:
var MailHelper = new MailHelper
{
Sender = sender, //email.Sender,
Recipient = useremail,
RecipientCC = null,
Subject = emailSubject,
Body = messageBody
};
MailHelper.Send();
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
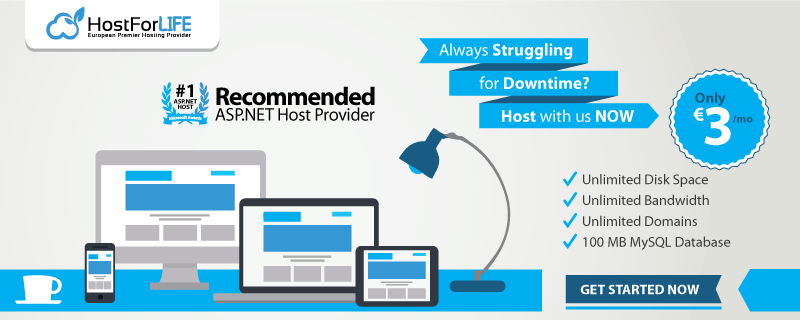