
March 30, 2016 23:25 by
Peter
Today, we will explain you about how to create and update cookie in ASP.NET MVC. An HTTP cookie (also called web cookie, Internet cookie, browser cookie or simply cookie), is a small piece of data sent from a website and stored in the user's web browser while the user is browsing. Every time the user loads the website, the browser sends the cookie back to the server to notify the user's previous activity. Cookies were designed to be a reliable mechanism for websites to remember stateful information (such as items added in the shopping cart in an online store) or to record the user's browsing activity (including clicking particular buttons, logging in, or recording which pages were visited in the past). Cookies can also store passwords and form content a user has previously entered, such as a credit card number or an address.
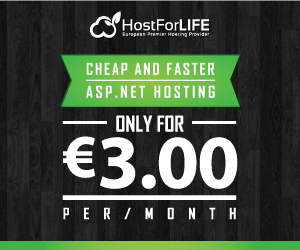
The output of the index.aspx runs over the Home Controller:
public ActionResult Index()
{
ViewData["Message"] = "Welcome to ASP.NET MVC!";
string cookie = "There is no cookie!";
if(this.ControllerContext.HttpContext.Request.Cookies.AllKeys.Contains("Cookie"))
{
cookie = "Yeah - Cookie: " + this.ControllerContext.HttpContext.Request.Cookies["Cookie"].Value;
}
ViewData["Cookie"] = cookie;
return View();
}
Here it is detected if a cookie exists and if yes than it will be out given.
These two Links guide you to the CookieController:
public class CookieController : Controller
{
public ActionResult Create()
{
HttpCookie cookie = new HttpCookie("Cookie");
cookie.Value = "Hello Cookie! CreatedOn: " + DateTime.Now.ToShortTimeString();
this.ControllerContext.HttpContext.Response.Cookies.Add(cookie);
return RedirectToAction("Index", "Home");
}
public ActionResult Remove()
{
if (this.ControllerContext.HttpContext.Request.Cookies.AllKeys.Contains("Cookie"))
{
HttpCookie cookie = this.ControllerContext.HttpContext.Request.Cookies["Cookie"];
cookie.Expires = DateTime.Now.AddDays(-1);
this.ControllerContext.HttpContext.Response.Cookies.Add(cookie);
}
return RedirectToAction("Index", "Home");
}
}
With the create method it´s quite simple to create a Cookie and lay it down into the response and afterwards it turns back to the Index View.
The remove method controls if a cookie exists and if the answer is positive the Cookie will be deleted directly.
Beware while deleting cookies:
This way to delete a cookie doesn´t work:
this.ControllerContext.HttpContext.Response.Cookies.Clear();
The cookie has to go back to the remove (like it is given in the Cookie Controller) and an expiry date should be given. I´m going to set it on yesterday so the browser has to refuse it directly.
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
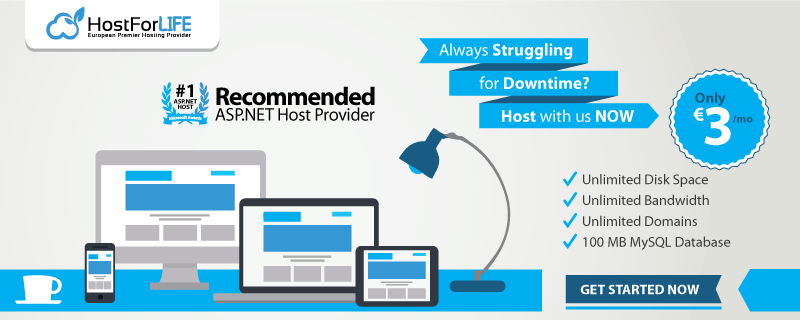