In this article, you will learn how use different actions to show a single view in ASP.NET MVC. It’s common situation in project development where you don’t want to create view for each and every action of certain controller. In this situation, we have to use concept of shared view. The solution is very simple, you just have to keep the view in shared folder. Like below:
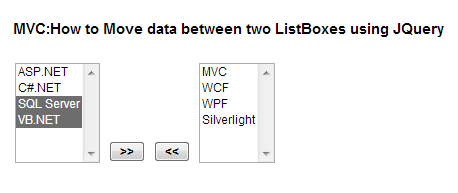
Now, The question is why need to keep in shared folder? The reason is when you run any controller, by default it check its own directory or shared directory. Every controller will look in shared directory, if it not available in it’s own directory. Let’s have a look on below code, let's create very simple controller class:
sing System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MVC3.Controllers
{
public class HomeController : Controller
{
//
// GET: /Home/
public ActionResult Action1()
{
ViewBag.Controller = "Action1";
return View("_Common");
}
public ActionResult Action2()
{
ViewBag.Controller = "Action2";
return View("_Common");
}
}
Both Action1() and Action2() is calling _Common view and as the view is placed in shared folder, both can able to access. Before calling to view we are assigning action name in ViewBag. From view we can detect which action has invoked it. Here is code for view:
<%@ Page
Language="C#"
Inherits="System.Web.Mvc.ViewPage<MVC3.Models.customer>"
%>
<!DOCTYPE html>
<html>
<head runat="server">
<title>_Common</title>
</head>
<body>
<div>
<% var ActionName = ViewBag.ActionName; %>
<% if (ActionName == "Action1")
{%>
This view is called from Action 1
<%}
else
{%>
This view is Called from Action 2
<%} %>
</div>
</body>
</html>
Here is the output:
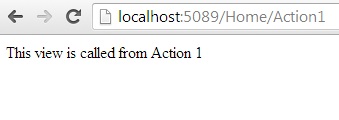
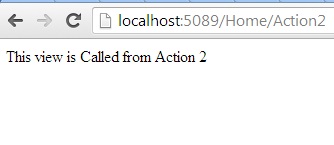
Now, the other question my show “Is it possible to call one view from different controller”? Yes, you can call. In below example, you will see how to do that.
You can call one view from different controller. This is your first controller Home1:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MVC3.Controllers
{
public class Home1Controller : Controller
{
public ActionResult Action()
{
ViewBag.Controller = "Home1";
return View("_Common");
}
}
}
And here is the output.
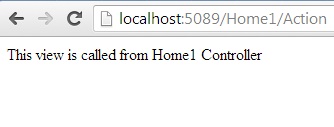
And for the Home2 controller:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MVC3.Controllers
{
public class Home2Controller : Controller
{
public ActionResult Action()
{
ViewBag.Controller = "Home2";
return View("_Common");
}
}
}
Here is the output:
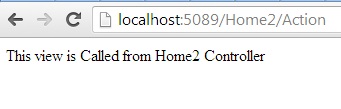
It’s clear that both Action() (they are in different controller) are calling same view.
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
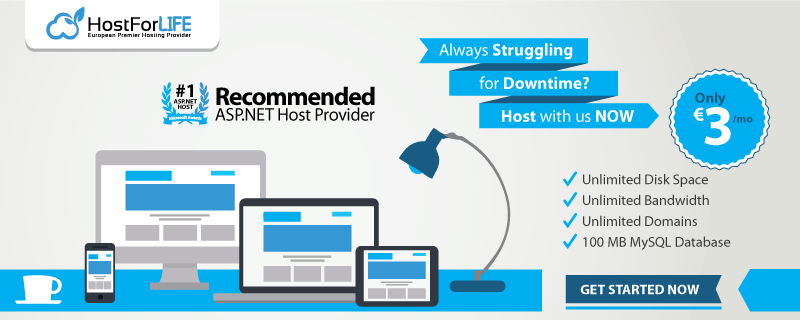