
February 28, 2020 11:15 by
Peter
In this post we will implement modal pop up to display the detailed information of user after clicking on detail anchor. So this is the view on which we are going to apply modal popup.
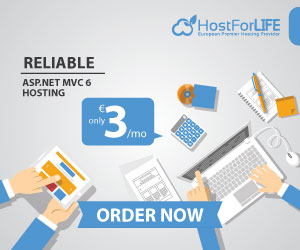
View code
Enumerable<CodeFirst.Models.FriendsInfo>
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
<p>
@Html.ActionLink("View All", "Index")
@using (Html.BeginForm("Search", "Home", FormMethod.Post))
{
<table>
<tr>
<td>
<input type="text" id="txtName" name="searchparam" placeholder="type here to search" />
</td>
<td>
<input type="submit" id="btnSubmit" value="Search" />
</td>
</tr>
</table>
}
</p>
<table class="table">
<tr>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.Mobile)
</th>
<th>
@Html.DisplayNameFor(model => model.Address)
</th>
<th></th>
</tr>
@foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.Mobile)
</td>
<td>
@Html.DisplayFor(modelItem => item.Address)
</td>
<td>
@*@Html.ActionLink("Edit", "Edit", new { id=item.Id }) |
@Html.ActionLink("Details", "Details", new { id=item.Id }) |
@Html.ActionLink("Delete", "Delete", new { id=item.Id })*@
<a href="javascript:void(0);" class="anchorDetail" data-id="@item.Id">Details</a>
</td>
</tr>
}
</table>
As we can see we have detail anchor, with class anchorDetail and with data-id, which will get the id of clicked anchor and show the corresponding data to modal (detail view) on screen.
We have an Action method Details(int id) which will return the partial view.
public ActionResult Details(int Id)
{
FriendsInfo frnds = new FriendsInfo();
frnds = db.FriendsInfo.Find(Id);
return PartialView("_Details",frnds);
}
Here we added a partial view for this purpose to show detail view when user click on detail anchor in the list.
View Code
@model CodeFirst.Models.FriendsInfo
<div>
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
<h4 class="modal-title" id="myModalLabel">FriendsInfo</h4>
</div>
<hr />
<dl class="dl-horizontal">
<dt>
@Html.DisplayNameFor(model => model.Name)
</dt>
<dd>
@Html.DisplayFor(model => model.Name)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Mobile)
</dt>
<dd>
@Html.DisplayFor(model => model.Mobile)
</dd>
<dt>
@Html.DisplayNameFor(model => model.Address)
</dt>
<dd>
@Html.DisplayFor(model => model.Address)
</dd>
</dl>
</div>
We have a div for modal pop-up.
<div id='myModal' class='modal'>
<div class="modal-dialog">
<div class="modal-content">
<div id='myModalContent'></div>
</div>
</div>
</div>
Here is the script for showing modal (partial view) on above div when user click on detail anchor. Here we used Ajax call for this purpose.
Script
@section scripts
{
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script src="~/Scripts/bootstrap.js"></script>
<script src="~/Scripts/bootstrap.min.js"></script>
<script>
var TeamDetailPostBackURL = '/Home/Details';
$(function () {
$(".anchorDetail").click(function () {
debugger;
var $buttonClicked = $(this);
var id = $buttonClicked.attr('data-id');
var options = { "backdrop": "static", keyboard: true };
$.ajax({
type: "GET",
url: TeamDetailPostBackURL,
contentType: "application/json; charset=utf-8",
data: { "Id": id },
datatype: "json",
success: function (data) {
debugger;
$('#myModalContent').html(data);
$('#myModal').modal(options);
$('#myModal').modal('show');
},
error: function () {
alert("Dynamic content load failed.");
}
});
});
//$("#closebtn").on('click',function(){
// $('#myModal').modal('hide');
$("#closbtn").click(function () {
$('#myModal').modal('hide');
});
});
</script>
}
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
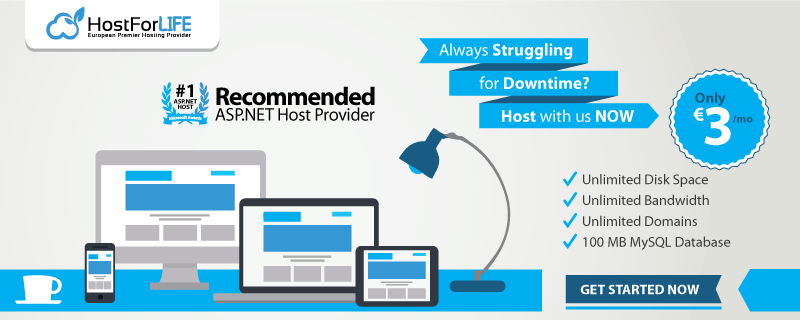