
September 15, 2023 06:57 by
Peter
ASP.NET MVC (Model-View-Controller) is a popular web development framework for creating strong and maintainable web applications. Model Binders are an important part of it. Model Binders play a critical role in mapping incoming HTTP requests to action method parameters, making it easier to work with client-side data. In this blog post, we will delve into the world of ASP.NET MVC Model Binders and present practical examples of how to use them.
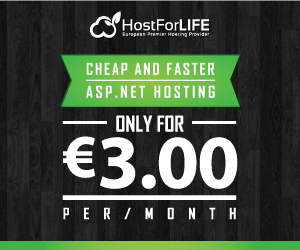
What Exactly Are Model Binders?
Model Binders are ASP.NET MVC components that are responsible for mapping data from numerous sources, such as form fields, query strings, route parameters, and JSON payloads, to action method parameters. They are critical in easing the process of taking user input and transforming it into highly typed objects that your program may use.
Here's a detailed breakdown of how Model Binders work:
- A user delivers an HTTP request to your ASP.NET MVC application, generally via a form submission or an API call.
- Routing: The MVC framework uses routing rules to identify which controller and action method should handle the request.
- Model Binders are useful in this situation. They collect data from the HTTP request and transfer it to the action method's arguments. The mapping is based on the names and types of parameters.
- Execution of Action Methods: After the data is bound to the action method parameters, the method is executed using the provided data.
- The action method processes the data and provides a response, which is returned to the client.
Now, let's look at some real-world examples of Model Binders.
Binding to Simple Types as an Example
Assume you have a simple HTML form with a single text input field named "username." You wish to capture the user's entered username.
public ActionResult Register(string username)
{
// Process the username
return View();
}
In this scenario, the Model Binder automatically binds the username parameter based on the name of the form input field.
Example 2: Complex Type Binding
Model Binders can also be used to tie complex types, such as custom classes, to action method parameters. Consider the following User class:
public class User
{
public string FirstName { get; set; }
public string LastName { get; set; }
}
You can bind an instance of the User class from a form submission as follows:
public ActionResult CreateUser(User user)
{
// Process the user object
return View();
}
The Model Binder will automatically populate the User object's properties using the submitted form data.
Example 3. Custom Model Binders
In some cases, you might need to implement custom Model Binders to handle complex scenarios. For example, if you want to bind data from a non-standard source or perform custom data transformation, you can create a custom Model Binder.
public class CustomBinder : IModelBinder
{
public object BindModel(ControllerContext controllerContext, ModelBindingContext bindingContext)
{
// Custom logic to bind the model
// Example: Read data from a cookie and populate the model
}
}
To use the custom Model Binder, you can decorate your action method parameter with the [ModelBinder] attribute:
public ActionResult MyAction([ModelBinder(typeof(CustomBinder))] MyModel model)
{
// Custom binding logic applied
return View();
}
ASP.NET MVC Model Binders are critical components that let you manage user input data in your online applications. They make it simple to convert HTTP request data to action method parameters, making it easier to work with user-supplied data. Model Binders provide a strong technique for streamlining data binding in your ASP.NET MVC applications, whether you're dealing with simple types or complex objects.
You can design more efficient and maintainable web apps with ASP.NET MVC if you understand how Model Binders function and use them effectively.