
July 30, 2020 13:16 by
Peter
Today, let me explain you how to handle multiple submit buttons in ASP.NET MVC 6. Sometimes you will need to handle multiple submit buttons on a similar form as as in the following picture.
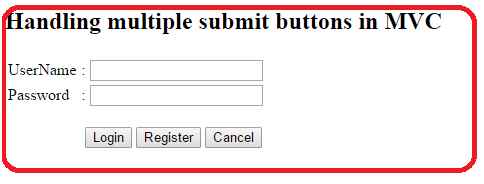
As you can see on the above picture, we've got the three buttons Login, Register and Cancel. Here every button has totally different functionality. in this way every submit button will post a form to the server but will provide totally different values of every button.
Make a controller with one action method that accepts other parameters, one is for the model and the other is for determining the status of the button click.
[HttpPost]
public ActionResult Index(Login model, string command)
{
if (command=="Login")
{
// do stuff
return RedirectToAction("Home");
}
else if (command=="Register")
{
// do stuff
ViewBag.msg = "You have Clicked Register button";
return View();
}
else if (command=="Cancel")
{
// do stuff
ViewBag.msg = "You have Clicked Cancel Button";
return View();
}
else
{
return View();
}
}
In the preceding code snippet, assume you clicked on the Login button, then the command parameter can have the values Login, null, null respectively. Create a View for the preceding controller.
@model MvcMultipleSubmitButtons.Models.Login
@{
ViewBag.Title = "Index";
}
<h2>
Handling multiple submit buttons in MVC </h2>
<h5 style="color: Red">@ViewBag.msg</h5>
<form action="Home/Index" id="myform" method="post" >
//here action name is Index, controller name is Home. So the action path is Home/Index
<table>
<tr>
<td>
UserName
</td>
<td>
:
</td>
<td>@Html.TextBoxFor(m => m.userName)
</td>
<td>
@Html.ValidationMessageFor(m => m.userName)
</td>
</tr>
<tr>
<td>
Password
</td>
<td>
:
</td>
<td>@Html.TextBoxFor(m => m.password)
</td>
<td>
@Html.ValidationMessageFor(m => m.password)
</td>
</tr>
</table>
<br/>
<div style="padding-left: 80px;">
<input type="submit" id="Login" value="Login" name="Command" title="Login" />
<input type="submit" id="Register" value="Register" name="Command" title="Register" />
<input type="submit" value="Cancel" name="Command" title="Cancel" />
</div>
</form>
You can declare the form tag in another way as within the following:
@using(Html.BeginForm("Index","Home",FormMethod.Post))
{
//here action name is Index, controller name is Home and form method is post.
}
Note: there's a relation between button name and action method parameter. for instance, the button name is “Command”, the action parameter name ought to be “command”.
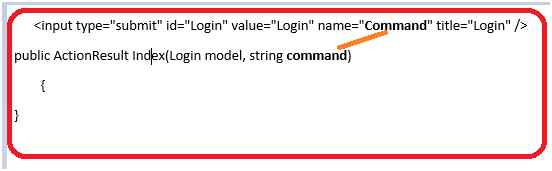
You can have different names for each button. So in that case you need to handle it as in the following:
<input type="submit" id="Login" value="Login" name="Command1" title="Login" />
<input type="submit" id="Register" value="Register" name="Command2" title="Register" />
<input type="submit" value="Cancel" name="Command3" title="Cancel" />
Controller
public ActionResult Index(Login model, string command1, string command2, string command3)
{
// here command1 is for Login, command2 is for Register and command3 is for cancel
}
Create a Model class with the name Login.
public class Login
{
public string userName { get; set; }
public string password { get; set; }
}
I hope it helps for you!
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
