Today, I will tell you about Dynamic Auto Complete in MVC for any Table of DataBase. With the following code, we can file record of product code on the basis of product name. Now write the code below:
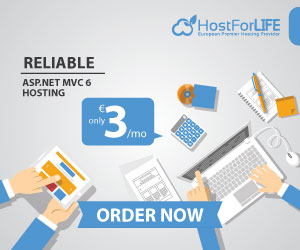
View
@model string < input type = "hidden"
id = "TableName_@Model"
value = "@Model" / > @Html.TextBox("txtCode_" + @Model)
@Html.TextBox("txtName_" + @Model) < script type = "text/javascript" > $(function ()
{
$('#txtName_@Model').autocomplete(
{
source: function (request, response)
{
alert("hi");
var id = $('#TableName_@Model').val();
$.ajax(
{
url: "/Common/AutocompleteName/" + id + "?name=" + $('#txtName_@Model').val(),
dataType: "json",
type: 'POST',
data:
{
name: request.term
},
success: function (data)
{
response(data);
}
});
},
autoFocus: true,
select: function (event, ui)
{
var id = $('#TableName_@Model').val();
var mData;
var unit;
$.ajax(
{
url: "/Common/GetCodeName/" + id,
type: 'POST',
data:
{
codeName: ui.item.value,
mPara: 'N'
},
success: function (_result)
{
// alert(_result);
mData = _result.UserName;
unit = _result.unitdata;
setTimeout(function ()
{
$('#txtCode_@Model').val(mData);
}, 1000);
$('#Description_@Model').val(mData);
$('#Units_@Model').html(unit);
}
});
},
minLength: 1
});
$('#txtCode_@Model').autocomplete(
{
source: function (request, response)
{
var id = $('#TableName_@Model').val();
$.ajax(
{
url: "/Common/AutocompleteCode/" + id + "?code=" + $('#txtCode_@Model').val(),
dataType: "json",
data:
{
code: request.term
},
success: function (data)
{
response(data);
},
type: 'POST'
});
},
autoFocus: true,
select: function (event, ui)
{
var id = $('#TableName_@Model').val();
var mData;
var unit;
$.ajax(
{
url: "/Common/GetCodeName/" + id,
type: 'POST',
data:
{
codeName: ui.item.value,
mPara: 'C'
},
success: function (_result)
{
unit = _result.unitdata;
mData = _result.UserName;
setTimeout(function ()
{
$('#txtName_@Model').val(mData);
}, 1000);
$('#Units_@Model').html(unit);
$('#Description_@Model').val(mData);
}
});
},
minLength: 1
});
}); < /script>
Controller-- -- -- -- -- -- -- -- -- -- -- --[HttpPost]
public JsonResult AutocompleteName(string id, string name)
{
var TblSet = Core.CoreCommon.GetTableData(id);
var output = TblSet.AsQueryable().ToListAsync().Result.ToList();
Dal.TFAT_WEBERPEntities context = new Dal.TFAT_WEBERPEntities();
return Json(from m in output where m.GetType().GetProperty("Name").GetValue(m).ToString().Contains(name) select m.GetType().GetProperty("Name").GetValue(m).ToString());
}
[HttpPost]
public JsonResult AutocompleteCode(string id, string code)
{
var TblSet = Core.CoreCommon.GetTableData(id);
var output = TblSet.AsQueryable().ToListAsync().Result.ToList();
return Json(from m in output where m.GetType().GetProperty("Code").GetValue(m).ToString().Contains(code) select m.GetType().GetProperty("Code").GetValue(m).ToString());
}
[HttpPost]
public ActionResult GetCodeName(string id, string codeName, string mPara)
{
var TblSet = Core.CoreCommon.GetTableData(id);
var output = TblSet.AsQueryable().ToListAsync().Result.ToList();
Dal.TFAT_WEBERPEntities context = new Dal.TFAT_WEBERPEntities();
string UserName = "";
string unitdata = "";
string Product = "";
if (mPara == "C")
{
var query = (from m in output where m.GetType().GetProperty("Code").GetValue(m).ToString().Contains(codeName) select m.GetType().GetProperty("Name").GetValue(m).ToString());
if (id == "ItemMaster")
{
var query1 = (from m in output where m.GetType().GetProperty("Code").GetValue(m).ToString().Contains(codeName) select m.GetType().GetProperty("Unit").GetValue(m).ToString());
var NewQuery = (from c in output where c.GetType().GetProperty("Code").GetValue(c).ToString().Contains(codeName) select c.GetType().GetProperty("Name").GetValue(c).ToString());
if (query1 != null)
{
unitdata = query1.First().ToString();
}
if (NewQuery != null)
{
Product = NewQuery.First().ToString();
}
}
if (query != null)
{
UserName = query.First().ToString();
}
}
else
{
var query = (from m in output where m.GetType().GetProperty("Name").GetValue(m).ToString().Contains(codeName) select m.GetType().GetProperty("Code").GetValue(m).ToString());
if (query != null)
{
UserName = query.First().ToString();
}
}
return Json(new
{
UserName,
unitdata,
Product
});
// return Content(UserName);
}
Common Class-- -- -- -- -- -- -- -- -- - public class CoreCommon
{
public static object GetTableObject(string TableName)
{
Type mType = BuildManager.GetType(string.Format("TFATERPWebApplication.Dal.{0}", TableName), true);
return System.Activator.CreateInstance(mType);
}
public static Type GetTableType(string TableName)
{
return BuildManager.GetType(string.Format("TFATERPWebApplication.Dal.{0}", TableName), true);
}
public static DbSet GetTableData(string tablename)
{
var mType = BuildManager.GetType(string.Format("TFATERPWebApplication.Dal.{0}", tablename), true);
TFAT_WEBERPEntities ctx = new TFAT_WEBERPEntities();
return ctx.Set(mType);
}
public static string GetString(string[] col)
{
StringBuilder sb = new StringBuilder();
foreach(string s in col)
{
sb.Append(s);
sb.Append(",");
}
return sb.ToString().Substring(0, sb.Length - 1);
}
}
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
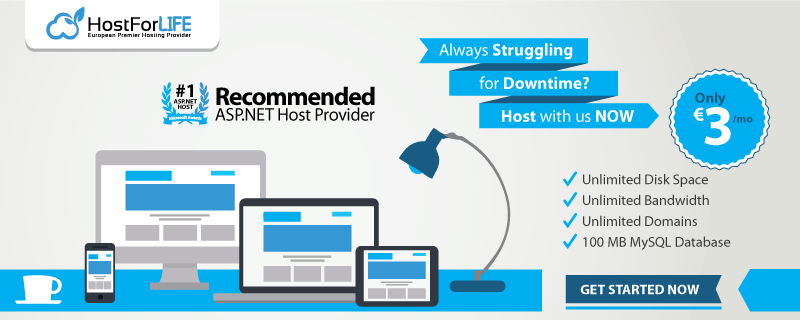