In today’s article we will look at an extremely useful feature. In many applications, the data we collect on the user interface needs to be converted to some different form before it can be stored in the data store and vice versa. This requires some mapping logic and it is mostly done using mapping classes and logic. Today, we will look at a ready to use Nuget package to do this mapping for us and make the process amazingly simple. We will apply this mapping in an ASP.NET Core MVC application.
Creating the .NET Core MVC application
We will start by creating an ASP.NET Core 3.1 MVC application using Visual Studio 2019 community edition as below,
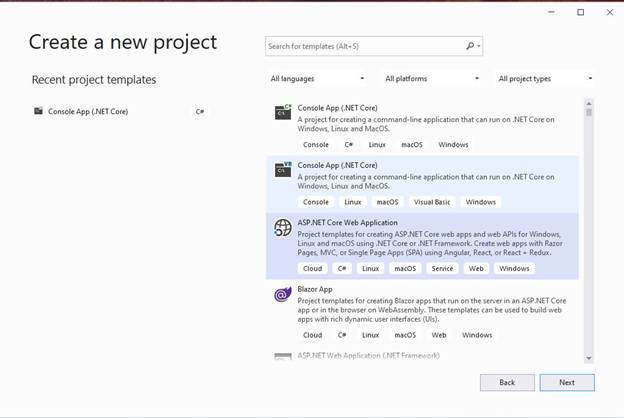
We select the ASP.NET Core web application and click “Next”
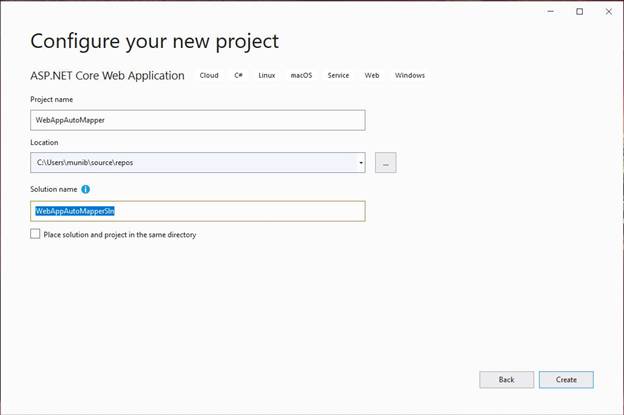
Next, we enter the project details and click “Create”
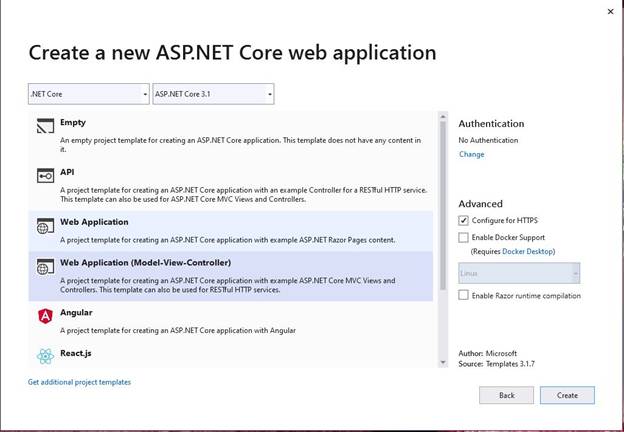
From the list of ASP.NET Core web applications, we select “Web Application (Model-View-Controller) and leave all other values as default.
We now have the ASP.NET Core MVC application created. The next step is to add the “AutoMapper” Nugget package as below,
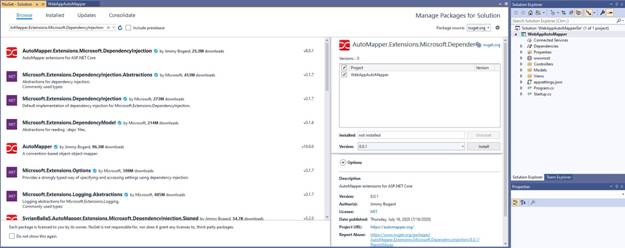
Remember to select the latest version.
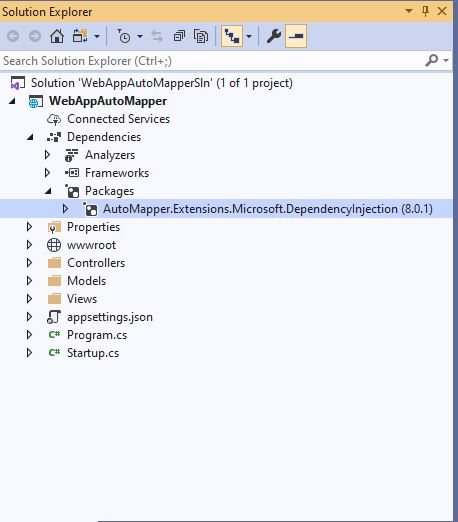
After that create three classes under the “Models” folder as below,
Employee.cs
namespace WebAppAutoMapper.Models {
publicclassEmployee {
publicstring FirstName {
get;
set;
}
publicstring LastName {
get;
set;
}
publicstring StreetAddress {
get;
set;
}
publicstring City {
get;
set;
}
publicstring Province {
get;
set;
}
publicstring Country {
get;
set;
}
publicstring Email {
get;
set;
}
publicstring Phone {
get;
set;
}
publicEmployee() {
FirstName = "Munib";
LastName = "Butt";
StreetAddress = "123 Street One";
City = "Toronto";
Province = "Ontario";
Country = "Canada";
Email = "[email protected]";
Phone = "+14161112222";
}
}
}
EmployeeDTO.cs
namespace WebAppAutoMapper.Models {
publicclassEmployeeDTO {
publicstring Name {
get;
set;
}
publicstring Address {
get;
set;
}
publicstring Email {
get;
set;
}
publicstring Phone {
get;
set;
}
}
}
And finally,AutoMap.cs
using AutoMapper;
namespace WebAppAutoMapper.Models
{
publicclassAutoMap : Profile
{
publicAutoMap()
{
CreateMap<Employee, EmployeeDTO>() // means you want to map from Employee to EmployeeDTO
.ForMember(d => d.Name, source => source.MapFrom(s => s.FirstName + " " + s.LastName))
.ForMember(d => d.Address, source => source.MapFrom(s => s.StreetAddress + ", " + s.City + ", " + s.Province + ", " + s.Country))
.ForMember(d => d.Phone, source => source.MapFrom(s => s.Phone))
.ForMember(d => d.Email, source => source.MapFrom(s => s.Email));
}
}
}
Here we see that we have an Employee class which has several properties. We also have an EmployeeDTO class which has fewer properties than the Employee class. Now, we want to map the values from the Employee class to the EmployeeDTO class using some mapping logic. This logic is implemented in the AutoMap.cs class which inherits from the Profile class.
Finally, we setup the AutoMap in the Startup.cs class in the “ConfigureServices” function as below,
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using AutoMapper;
using WebAppAutoMapper.Models;
namespace WebAppAutoMapper
{
publicclassStartup
{
publicStartup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
publicvoid ConfigureServices(IServiceCollection services)
{
services.AddAutoMapper(c => c.AddProfile<AutoMap>(), typeof(Startup));
services.AddControllersWithViews();
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
publicvoid Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
}
}
Now, all the plumbing is in place and we can use the mapping as below in the Home Controller,
using System.Diagnostics;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Logging;
using WebAppAutoMapper.Models;
using AutoMapper;
namespace WebAppAutoMapper.Controllers
{
publicclassHomeController : Controller
{
privatereadonly ILogger<HomeController> _logger;
privatereadonly IMapper _mapper;
publicHomeController(ILogger<HomeController> logger, IMapper mapper)
{
_logger = logger;
_mapper = mapper;
}
public IActionResult Index()
{
Employee emp = new Employee();
var empDTO = _mapper.Map<EmployeeDTO>(emp);
return View();
}
public IActionResult Privacy()
{
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
}
We can put a breakpoint at the “return View();” line in the Index function and see the Employee DTO values as below,
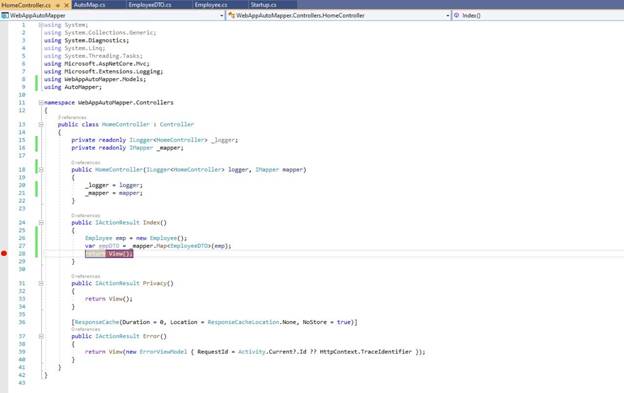
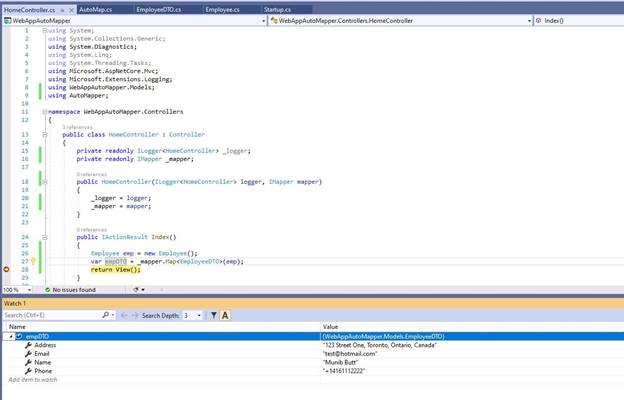
Here we see that the empDTO object has the values extracted and mapped from the Employee object.
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
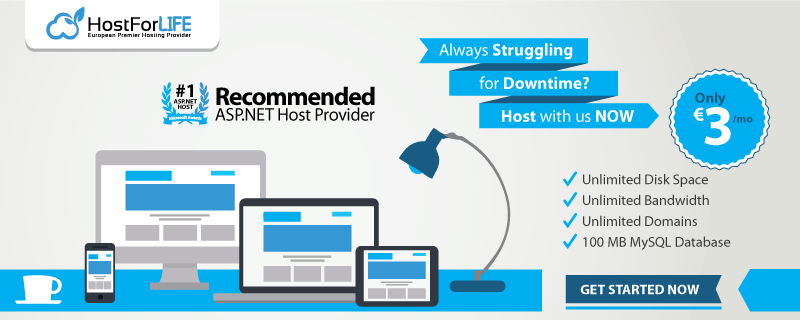